Behat Testing
admin
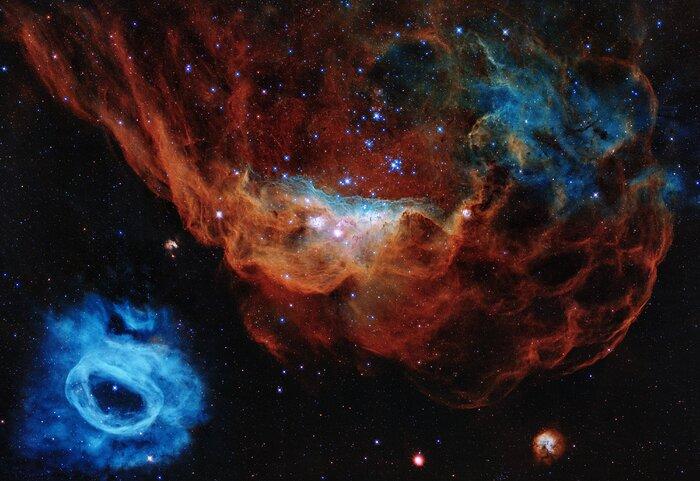
Create a custom context definition.
<?php
use Behat\Behat\Context\Context;
use Behat\Gherkin\Node\PyStringNode;
use Behat\Gherkin\Node\TableNode;
use Drupal\Core\Url;
use Behat\Mink\Exception\ExpectationException;
/**
* Defines available tests in a context.
*/
class MyContext extends \Drupal\DrupalExtension\Context\RawDrupalContext {
protected $output;
/**
* Make MinkContext available.
* @var \Drupal\DrupalExtension\Context\MinkContext
*/
private $minkContext;
/**
* Make DrupalContext available.
* @var \Drupal\DrupalExtension\Context\DrupalContext
*/
private $drupalContext;
/**
* Initializes context.
*
* Every scenario gets its own context instance.
* You can also pass arbitrary arguments to the
* context constructor through behat.yml.
*/
public function __construct() {
}
/**
* @Given I am logged in as user :name
* Thanks Jeff! https://www.jeffgeerling.com/blog/2018/logging-existing-user-behat-test-drupal-extension
*/
public function iAmLoggedInAsUser($name) {
$domain = $this->getMinkParameter('base_url');
// Pass base url to drush command.
$uli = $this->getDriver('drush')->drush('uli', [
"--name '" . $name . "'",
"--browser=0",
"--uri=$domain",
]);
// Trim EOL characters.
$uli = trim($uli);
// Log in.
$this->getSession()->visit($uli);
}
/**
* @Then :haystack text should contain :needle
*/
protected function assertTxtContains($haystack, $needle, $message = null) {
if (strpos($haystack, $needle) === FALSE) {
if (is_null($message)) {
$message = sprintf('The string "%s" was not found.', $expected);
}
throw new ExpectationException($message, $this->getSession());
}
}
/**
* @Given I have a file named :filename
*/
public function iHaveAFileNamed($filename) {
touch($filename);
}
/**
* @When I run :command
*/
public function iRun($command) {
$this->output = shell_exec($command);
}
/**
* @Then I want to see :string in the output
*/
public function iShouldSeeInTheOutput($findme) {
$this->assertTxtContains((string) $this->output, $findme, sprintf('Did not see "%s" in output "%s"', $findme, $this->output));
}
}